Your First HTML Page
If you use a local editor like VS Code or one of the more advanced online editors like CodeSandbox or Stackblitz there are a few steps you need to take for creating a web page:
-
Open the editor
-
Create a new file and save it as an HTML file
-
Write HTML Code
-
Open the HTML file in a browser
After that, you can make changes in the HTML code and refresh the page in your browser to see what changes.
Hands-On Instructions For Visual Studio Code
I am only explaining this in detail for Visual Studio Code (VS Code) and not for every possible editor in existence, but these steps shouldn't be that much different for other editors. I am sure, you'll figure it out yourself. If in need of help, remember Google is your friend and AI chatbots like ChatGPT, Perplexity AI and Phind as well. š
1. Open VS Code
I hope that is self-explanatory.
Click on "New File", then enter index.html
in the panel at the top of the screen to give your new file a name. The .html
is important so that VS Code knows, that you intend to write HTML code in the new file. VS Code will then give keywords a certain color which is called syntax highlighting.
It's recommended to call the file index.html
because it is the main HTML file, i.e. the start page of your website. The name is also related to the way a page is accessed via URLs.
2. Option B: Create a new text file and save as HTML later on
There are also multiple ways to create a text file in VS Code without defining the file type upfront:
- Via the file menu in the top left corner: "File" > "New Text File"
- press the keyboard shortcut "Ctrl" + "n".
- double click in the empty tab bar next to the "Welcome" Tab
In such a case, neither a file type nor a programming language is defined, yet. So, there is no syntax highlighting, meaning the whole text is displayed in a plain color, e.g. white.
You can either start writing HTML code (step 3.) or save the file directly as HTML. Whatever you do, at some point you have to save the file with the file extension .html
.
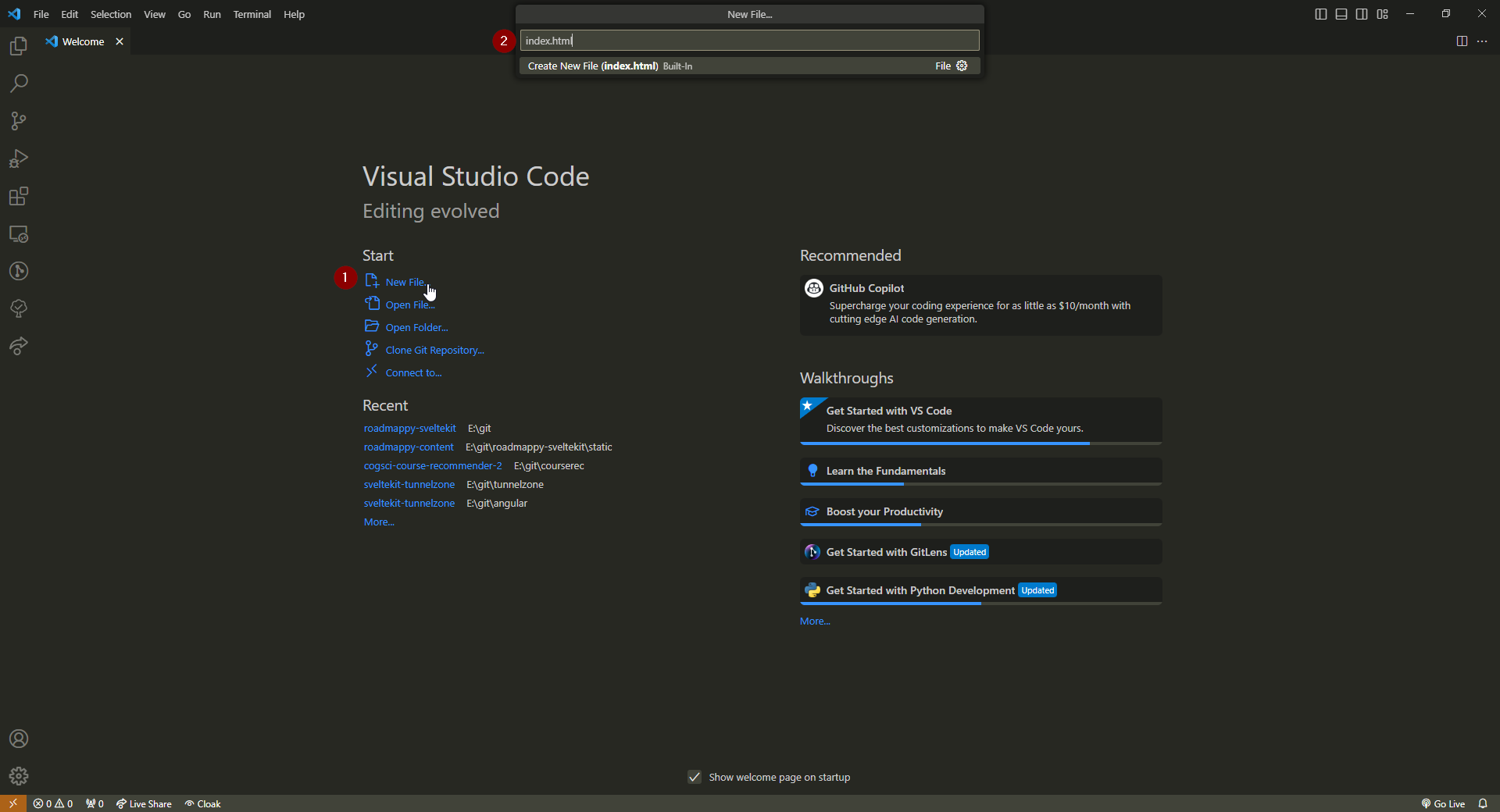
Usually, you would open a folder for your web project where your HTML files, images and some other files would be organized. But organizing a web or software project is an topic in itself which will be covered at an appropriate time.
3. Type HTML code in your file
A good first example for you to try is to type a heading tag.
<h1>Hello World</h1>
Just to give you a quick moment of success, we are leaving out the typical <html>
, <head>
, <body>
HTML skeleton for now. These elements are not mandatory, because modern browsers add them automatically; often times correctly but not always. A few more details further below...
Then, save the file via the menu: "File > Save" or by pressing the keyboard shortcut "Ctrl"+"s".
4. Open your index.html
file in a browser
The easiest way to open your file in a browser is to go to where you saved it using Finder (MacOS) or Windows Explorer (Windows). Then you can either double-click the file to open it in your default browser, or drag and drop it into a browser window that you already opened beforehand.
If you wrote everything correctly a big black 'Hello World' text should display on the white background! š„³š
If you don't remember where you saved your file, you can open the directory of your file via VS Code. You can right click on the tab of the index.html
tab and click "Reveal in File Explorer" (on Windows) or "Reveal in Finder" (on MacOS).
Add The HTML Skeleton
In theory, you don't need to add a <html>
, <head>
, <body>
tag etc., because the browser adds these elements automatically, but without this structure your code is a bit ambiguous which can lead to a wrong interpretation. Therefore, I always recommend to still add this kind of HTML skeleton yourself, to prevent any wrong interpretations of a browser).
You could check out how the browser did actually interpret your code by opening the browser developer tools while your web page is open. How to open the dev tools is explained on the page Challenge: Analyze The Code Of Public Websites.
Below is the complete code of an exemplary web page that you can use as a starting point and a reference for typing.
In general it is recommended to type everything yourself and not use copy and paste. As a beginner trying to type by yourself will help you remember the code and its keywords better. Even being precise about small details like the correct special characters (<
, >
, /
and "
) as well as adding space ā
at the right location is important! By writing everything yourself, you will inevitably make mistakes. Fixing these occasional mistakes are an important part of your learning journey!
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Website</title>
</head>
<body>
<h1>Hello World</h1>
<p>Let's start with a sentence. Here is another one.</p>
<p>This sentence is alone in this paragraph</p>
</body>
</html>
Don't forget to save your file and then refresh your browser.
The Edit, Save And Refresh Cycle
After you have your general HTML skeleton set up, you can get creative and add more content and elements.
For example, you could try adding an unordered list of items:
<ul>
<li>Apples</li>
<li>Bananas</li>
<li>Oranges</li>
</ul>
While developing your are basically following this cyclic workflow:
- Making changes to your code
- Saving
- Refreshing the browser
- Repeat
It's easy to forget either saving or refreshing. So, if that is a regular problem for you, can activate a setting in VS Code to automatically save after a defined amount of second. And I can highly recommend the LiveServer extension for VS Code which automatically refreshes the content of your web page when the respective file is saved.
Challenges: Play Around And Find Out
You could add some more tags from one of these reference sites or try out the challenges below:
What happens when you put extra spaces or line breaks in your document? Below is some code that you can copy to try it out.
You can read a detailed explanation here MDN about Whitespace
<p>Did you know that
HTML
reduces multiple spaces and
other whitespace characters into a single whitespace character?</p>
In VS Code in the bottom in the bar at the bottom, do you see the small text "HTML" or "Plain Text" in the right bottom corner? What happens to your HTML code when you press it and switch between the options "XML", "HTML" or "Plain Text"?
The button "Select Language Mode" is switching the programming language that VS Code uses for syntax highlighting. So, when you select "Plain Text" your code or whatever you wrote will be displayed in a plain color, e.g. white. When you select the appropriate language that matches the programming language of the code you wrote, programming keywords will be highlighted in a distinct color, depending on the particular keyword.
Next Steps
Next, you could